Operating System Segmentation: A Complete Guide
Updated on : 21 April 2025
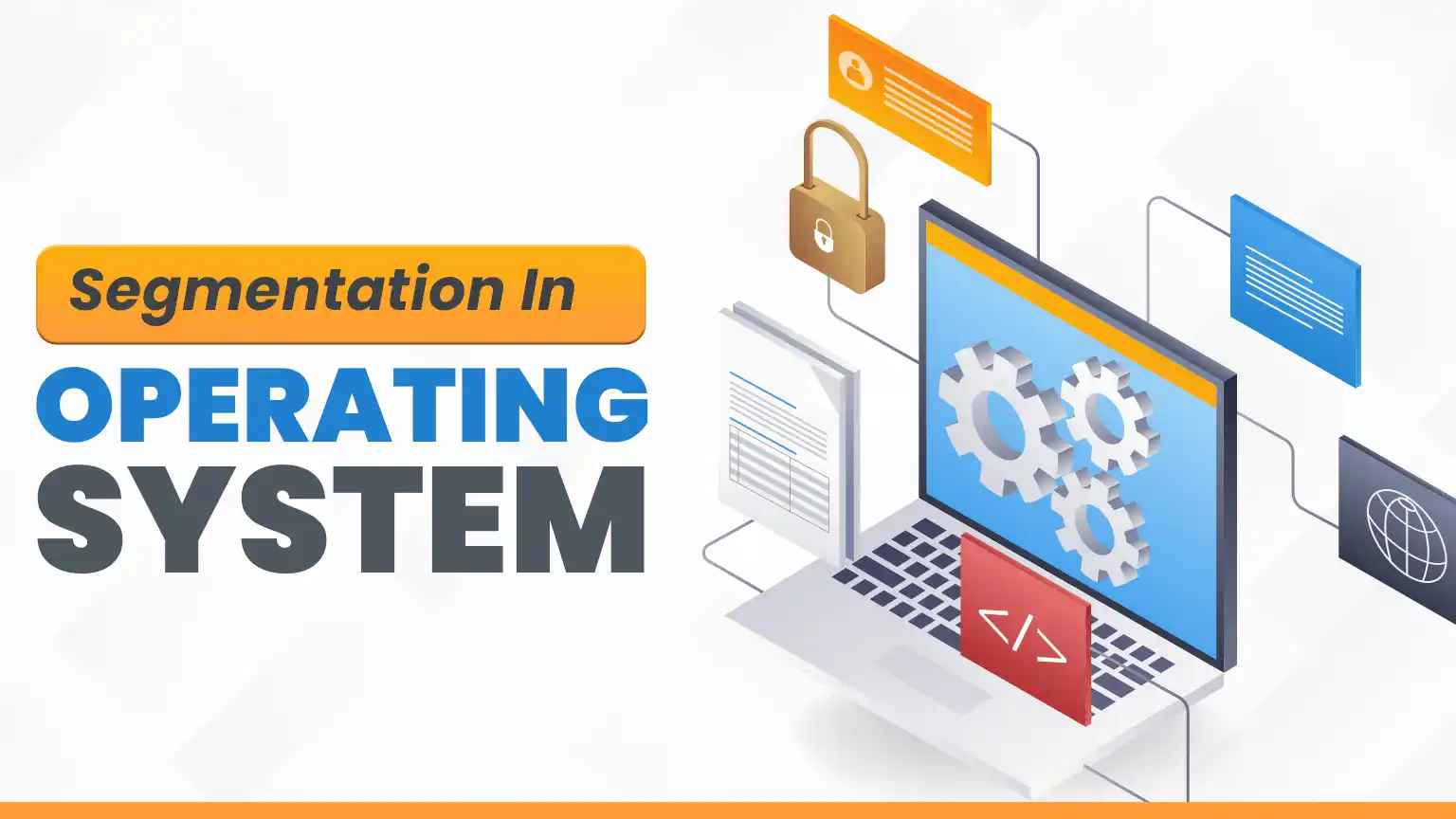
Image Source: google.com
Table Of Contents
Introduction
Segmentation is a memory management technique in operating systems that divides a program's memory into distinct segments, each representing a logical unit such as code, data, or stack.
- Unlike paging, which splits memory into fixed-size blocks, segmentation uses variable-sized segments that correspond more closely to the way programmers and users think about their programs.
- Each segment is a contiguous block of memory but segments themselves can be scattered throughout the physical memory, making segmentation a non-contiguous memory allocation method.
A segment can represent:
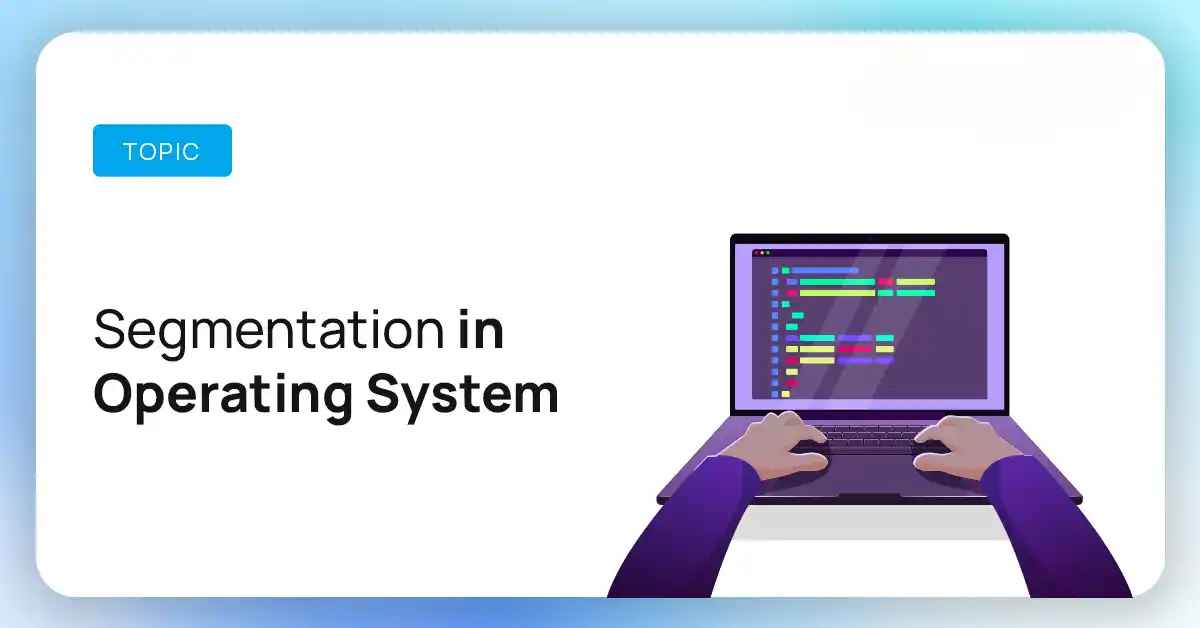
Image Source: google
1. A function or procedure
→ Code block (logical grouping of instructions that perform a task)
2. An array or data block
→ Data structure (collection of elements stored in memory)
3. The stack
→ Memory structure (used for function calls, local variables, etc.)
4. The symbol table
→ Compiler data structure (stores information about identifiers like variables, functions, etc.)
5. Any other logical grouping within a program
→ Could refer to modules, namespaces, or classes (used for organization and encapsulation)
For Cloud Services with Hexadecimal Software
Accelerate your digital transformation with Hexadecimal Software’s intelligent cloud service solutions
How Segmentation Works in OS
Segmentation is a memory management technique where a program is divided into different logical segments Each segment represents a logical unit (not just fixed-size blocks like in paging), and segments can be of different lengths.
Segmentation Overview
Concept | Description |
---|---|
Segmentation | Memory management technique that divides programs into logical segments |
Common Segments | Code, Data, Stack, Heap |
Purpose | Logical separation, access control, easier protection |
Logical vs Physical Address
Type | Description | Example Format |
---|---|---|
Logical Address | Generated by CPU, a pair of (Segment Number, Offset) | (1, 50) |
Physical Address | Actual memory location calculated using segment table | Base + Offset = 2000 + 50 = 2050 |
Segment Table Structure
Field | Description |
---|---|
Segment # | Identifier for each segment |
Base | Starting physical address of the segment |
Limit | Length (size) of the segment |
Segment # | Base Address | Limit (Size) |
---|---|---|
0 | 1000 | 500 |
1 | 2000 | 1000 |
2 | 5000 | 300 |
Address Translation
Step | Description |
---|---|
1 | CPU provides logical address (Segment Number, Offset) |
2 | Segment table is checked for base and limit |
3 | If Offset < Limit → OK, else → Segmentation Fault |
4 | Physical Address = Base + Offset |
Logical Address | Segment Base | Offset | Limit | Physical Address | Valid? |
---|---|---|---|---|---|
(1, 50) | 2000 | 50 | 1000 | 2050 | ✅ Yes |
(2, 350) | 5000 | 350 | 300 | - | ❌ No (Fault) |
For DevOps Services with Hexadecimal Software
Empowering seamless integration and deployment with Hexadecimal Software’s DevOps excellence.
Types of Segmentation
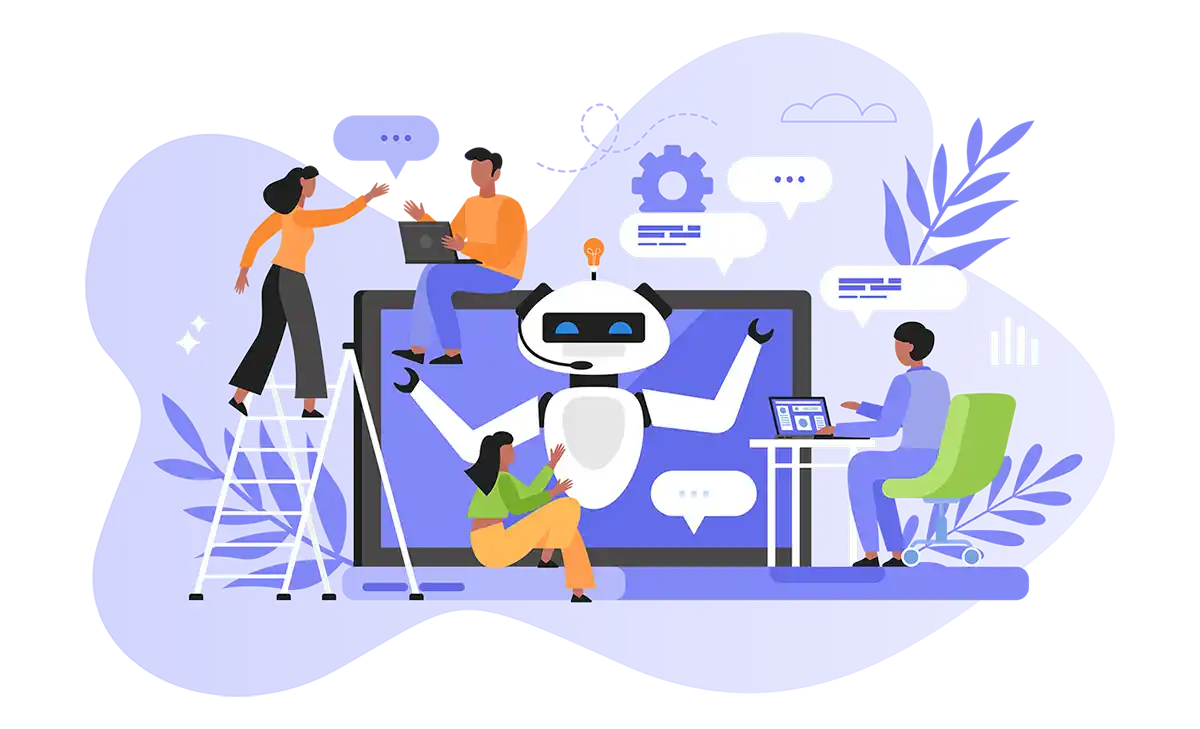
Image Source: google
1. Code Segment (Text Segment)
Purpose: Stores the executable instructions of a program.
Characteristics:
-
Usually marked as read-only to prevent accidental changes.
-
Shared among processes to save memory if they run the same code.
2. Data Segment
Purpose: Contains global and static variables.
Types:
-
Initialized Data Segment: Variables with assigned values.
-
Uninitialized Data Segment (BSS): Variables declared but not initialized.
Behavior: Loaded into memory at program startup and remains throughout execution.
3. Stack Segment
Purpose: Used for function call management.
Stores:
-
Local variables
-
Function parameters
-
Return addresses
Structure: Follows LIFO (Last In, First Out) principle.
- Grows/shrinks dynamically during function calls and returns.
4. Heap Segment (Bonus)
Purpose: Used for dynamic memory allocation at runtime.
Example: Memory allocated using new in Java or malloc() in C.
Behavior: Grows upward and must be managed manually or with garbage collection.
Segmentation vs Paging
Aspect | Segmentation | Paging |
---|---|---|
Memory Division | Divides memory into logical segments | Divides memory into fixed-size pages |
Size | Segments are of variable size | Pages are of fixed size |
Unit of Addressing | Segment number + offset | Page number + offset |
View of Memory | Logical (based on program structure) | Physical (uniform memory blocks) |
Fragmentation | Causes external fragmentation | Causes internal fragmentation |
Access Speed | Slightly slower due to variable sizes | Faster due to fixed-size pages |
Use Case | Better for modular programs (e.g., with functions, objects) | Efficient for OS-level memory management |
Protection & Sharing | Easy to provide protection per segment | Harder to protect individual logical structures |
Complexity | More complex to implement | Simpler implementation with fixed size |
Advantages and Disadvantages
Advantages | Disadvantages |
---|---|
Supports modularity (e.g., functions, objects) | Leads to external fragmentation |
Logical division of programs improves clarity | Variable segment sizes make memory management complex |
Allows independent growth of segments | Requires complex hardware for segment tables |
Enables better protection and access control | Slower access compared to paging in some cases |
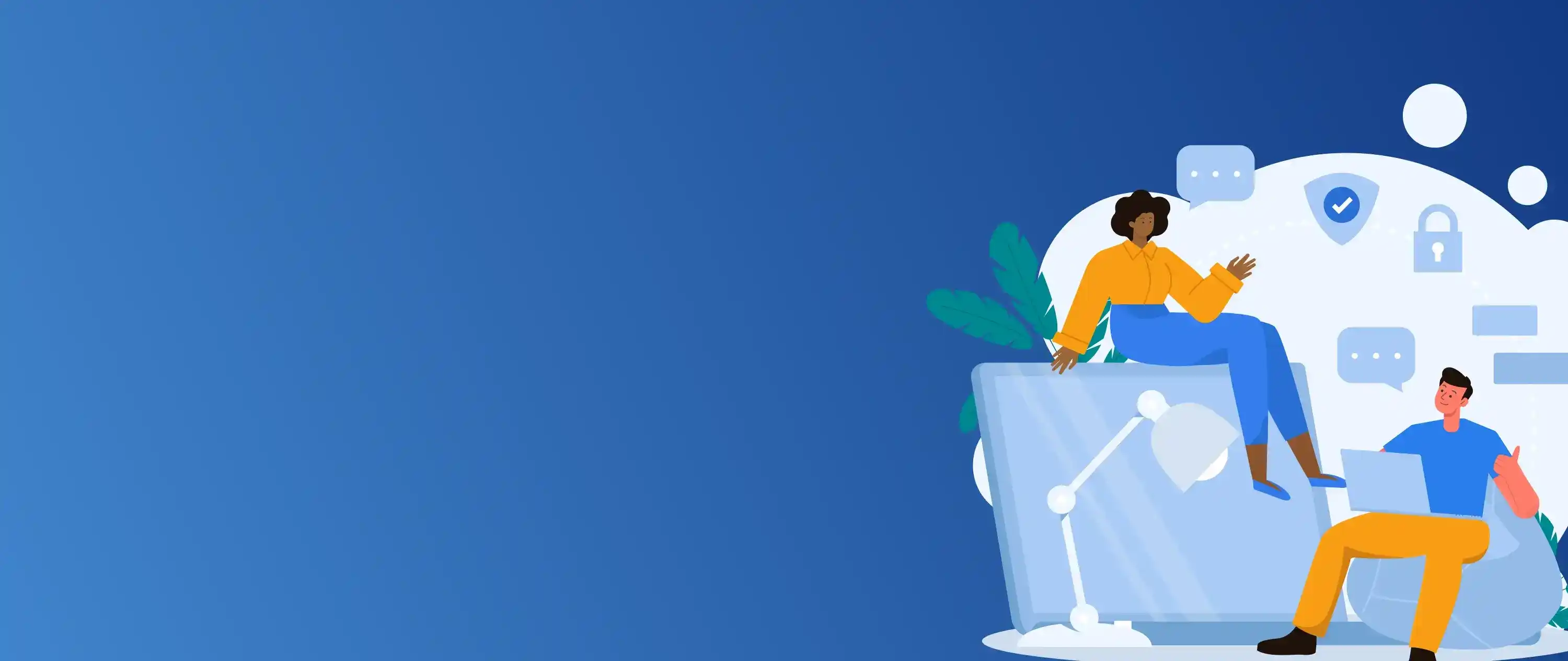
Looking to integrate Google Cloud solutions into your business?
Fragmentation in Segmentation
Fragmentation Type | Description | Handling |
---|---|---|
External | Scattered free memory makes large allocations hard. | Compaction, Segmentation with Paging |
Internal | Unused space within allocated segments (rare). | Less common in segmentation. |
Real-world Examples
Segment Type | Description | Example |
---|---|---|
Code Segment | Contains executable instructions | Functions like `openFile()`, `saveFile()` |
Data Segment | Stores global and static variables | Global variable `filePath = "/user/documents/file.txt"` |
Stack Segment | Used for local variables and function calls | Local variables like `char buffer[1024]` in `openFile()` |
Heap Segment | Manages dynamic memory allocation | Memory allocated for large document text storage |
Segmentation Diagrams
Segment | Description |
---|---|
Code Segment | Contains executable instructions |
Data Segment | Holds global and static variables |
Heap Segment | Dynamically allocated memory |
Stack Segment | Local variables and function calls |
Unused Memory | Free space or fragmented memory |
Code Segment
What it does: Stores the program’s compiled instructions — the actual code the CPU executes.
Includes: Functions, loops, conditionals, and other executable instructions.
Why it matters: It is usually marked read-only to prevent accidental modification, enhancing security and stability.
Data Segment
What it does: Holds global and static variables that are initialized or uninitialized.
Includes: Things like int counter = 0; or static buffers.
Why it matters: These variables exist throughout the program’s lifetime and need a stable, accessible memory location.
Heap Segment
What it does: Provides memory for dynamic allocation at runtime.
Includes: Memory allocated using functions like malloc() in C or new in Java.
Why it matters: The heap grows as needed (upward in most systems), offering flexibility for handling varying memory needs (e.g., dynamic arrays, objects).
Stack Segment
What it does: Stores data related to function calls.
Includes: Function call records (activation records), local variables, and return addresses.
Why it matters: The stack is organized and follows the Last-In-First-Out (LIFO) structure, which is ideal for tracking active functions.
Unused Memory
What it does: Represents free or fragmented space between segments.
Includes: Gaps that can result from segment growth/shrinkage or memory allocation patterns.
Why it matters: This space can lead to external fragmentation, which may require compaction to optimize memory usage.
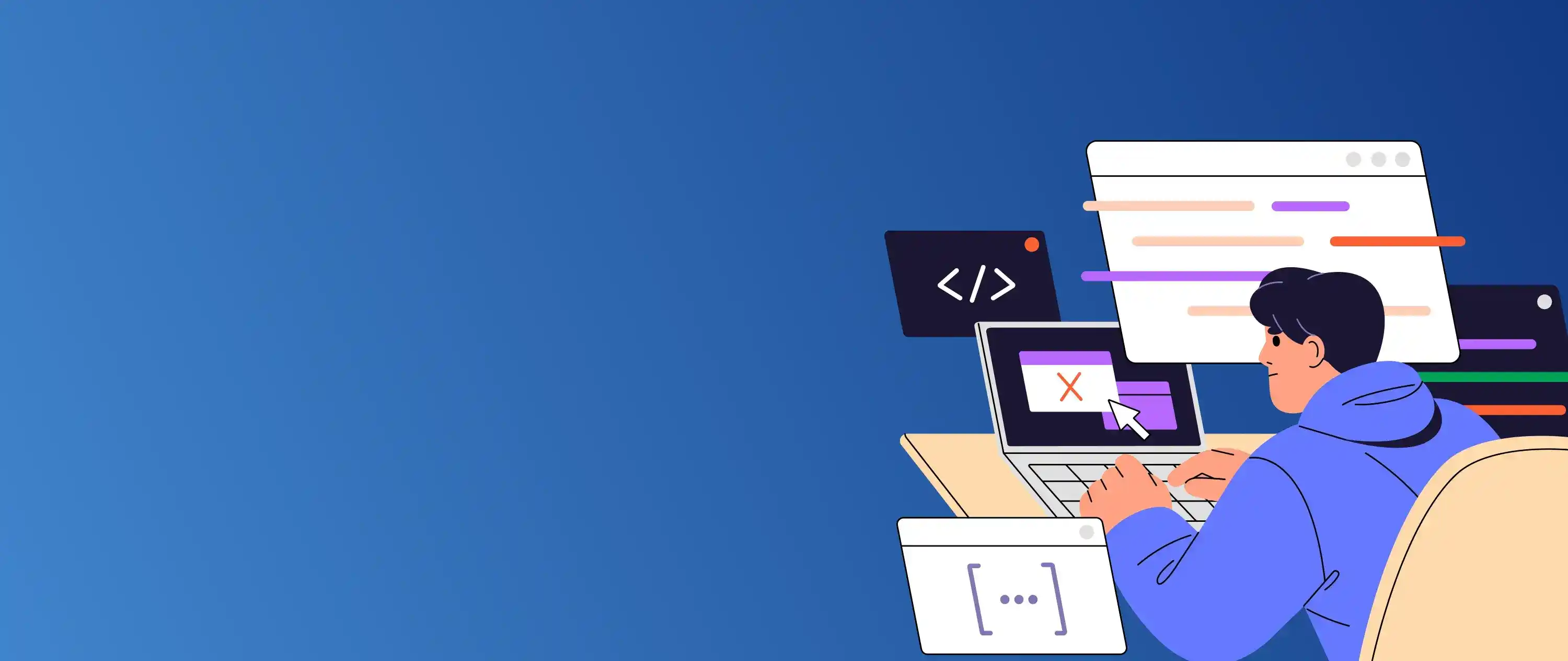
What if virtual became unforgettable with Hexadecimal Software?
Future Trends and Innovations in Segmentation
Trend / Innovation | Description |
---|---|
Hybrid Memory Models | Combining segmentation with paging for better performance and flexibility (e.g., x86 architecture). |
Enhanced Security | Isolating segments to prevent attacks like buffer overflows (e.g., ARM's MTE). |
Smarter OS & Compilers | AI-driven compilers optimizing memory layouts to reduce fragmentation and access time. |
Dynamic Segment Resizing | Support for growing or shrinking segments at runtime to adapt to memory needs. |
Virtualization Support | Segment-based isolation in containers/VMs to improve security in cloud environments. |
AI-Driven Management | Machine learning predicts memory usage and adjusts segment allocation dynamically. |
Conclusion
Segmentation remains a vital memory management technique that offers logical separation and protection of program components. It enhances modularity, simplifies access control, and supports efficient memory usage. Despite challenges like fragmentation, it is often combined with paging in modern systems. As OS designs evolve, segmentation continues to influence secure and optimized memory architectures.
🚀Connect With Us
FAQs
Q.1. What is segmentation in OS?
A : A memory management technique that divides programs into logical segments.
Q.2. How does segmentation differ from paging?
A : Segmentation uses variable-sized logical units, while paging uses fixed-sized blocks.
Q.3. What is a logical address in segmentation?
A : An address represented as a pair: (Segment Number, Offset).
Q.4. What causes a segmentation fault?
A : Accessing memory outside the segment’s defined limit.
Q.5. What are the main benefits of segmentation?
A : Modularity, protection, logical organization, and flexible memory usage.
Q.6. What is external fragmentation in segmentation?
A : Unused memory between segments that can’t be easily allocated.
Q.7. Is segmentation used in modern systems?
A : Yes, often in hybrid models with paging for optimized memory handling.