Java Thread Lifecycle Complete Beginners Guide
Updated on : 21 April 2025
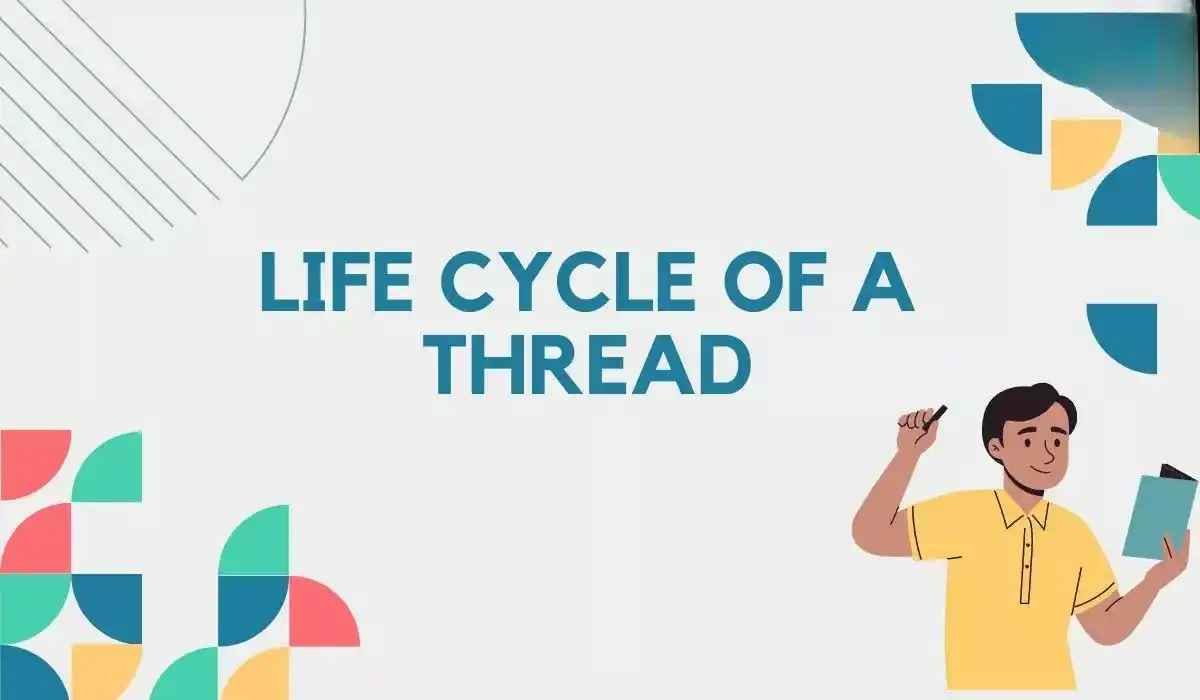
Image Source: google.com
Table Of Contents
- 1. Introduction
- 2. Thread Class and Runnable Interface
- 3. Life Cycle Transitions (State Changes)
- 4. Creating a Thread (New State)
- 5. Starting a Thread (Runnable State)
- 6. Running State Thread Execution
- 7. Blocked State Resource Contention
- 8. Waiting vs Timed Waiting
- 9. Thread.sleep(), join(), and yield() Methods
- 10. Thread Synchronization and Locks
- 11. Thread Priority and Scheduling
- 12. Dead State Thread Termination
- 13. Thread Management Best Practices
- 14. FAQs
Table Of Contents
Introduction
In Java, threads live an exciting life! 🧵 From being born (New 👶), getting ready (Runnable 🏃), springing into action (Running ⚡), sometimes getting stuck (Blocked 🚧), and finally retiring (Dead ⚰️) — each stage shows how Java keeps apps smooth and multitasking sharp
Thread Class and Runnable Interface
🧵 Using the Thread
Class
- You create a class that extends
Thread
. - Override the
run()
method with the code that the thread should execute. - Start the thread using
start()
.
class MyThread extends Thread {
public void run() {
System.out.println("Thread is running...");
}
}
public class Main {
public static void main(String[] args) {
MyThread t = new MyThread();
t.start(); // Starts the thread
}
}
🧩 Using the Runnable
Interface
- You create a class that implements
Runnable
. - Pass the instance to a
Thread
object. - Start the thread using
start()
.
class MyRunnable implements Runnable {
public void run() {
System.out.println("Thread is running...");
}
}
public class Main {
public static void main(String[] args) {
Thread t = new Thread(new MyRunnable());
t.start(); // Starts the thread
}
}
Life Cycle Transitions (State Changes)
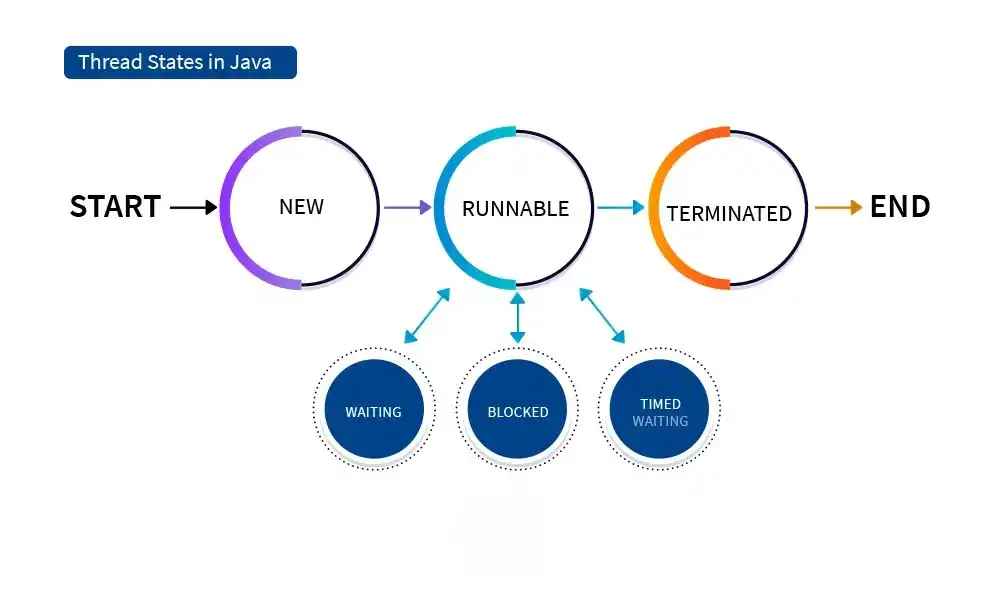
Image Source: google
From State | To State | Cause of Transition |
---|---|---|
New | Runnable | Thread.start() is called |
Runnable | Running | Thread is selected by CPU scheduler |
Running | Blocked/Waiting | Thread waits for a resource or lock |
Blocked/Waiting | Runnable | Resource becomes available or notified |
Running | Terminated | Thread completes or is forcefully stopped |
Runnable | Terminated | Thread is killed before it runs |
Creating a Thread (New State)
When a thread is created in Java, it enters the New state. This means the thread object has been instantiated but has not started executing yet.
There are two main ways to create a thread:
✅ 1. Extending the Thread
class
class MyThread extends Thread {
public void run() {
System.out.println("Thread is running...");
}
}
public class Main {
public static void main(String[] args) {
MyThread t = new MyThread(); // Thread is created (New State)
// t is now in New state
}
}
✅ 2. Implementing the Runnable
interface
java
class MyRunnable implements Runnable {
public void run() {
System.out.println("Thread is running...");
}
}
public class Main {
public static void main(String[] args) {
Runnable r = new MyRunnable();
Thread t = new Thread(r); // Thread is created (New State)
// t is in New state
}
}
Starting a Thread (Runnable State)
Once a thread is created (New state), calling the start()
method moves it to the Runnable state. This means the thread is ready to run but waiting for CPU time to be scheduled by the thread scheduler.
✅ Example Runnable State
class MyThread extends Thread {
public void run() {
System.out.println("Thread is running...");
}
}
public class Main {
public static void main(String[] args) {
MyThread t = new MyThread(); // New state
t.start(); // Now in Runnable state
}
}
🔄 What happens in Runnable state?
- The thread is now eligible to run, but it does not start immediately.
- The Thread Scheduler decides when the thread actually gets CPU time to move into the Running state.
- It may stay in Runnable state for some time depending on CPU availability and thread priority.
Running State Thread Execution
✅ Example Running State
class MyThread extends Thread {
public void run() {
System.out.println("Thread is running..."); // This runs in Running state
}
}
public class Main {
public static void main(String[] args) {
MyThread t = new MyThread(); // New
t.start(); // Runnable → Running (when CPU picks it)
}
}
⚙️ Key Points:
- Only one thread per core can be in the Running state at a time.
- Other threads remain in Runnable, waiting for their turn.
- The actual switch from Runnable to Running is controlled by the JVM thread scheduler, not the programmer.
Blocked State Resource Contention
A thread enters the Blocked state when it tries to access a resource (like an object or a section of code) that is locked by another thread. It remains blocked until the resource becomes available.
When does a thread get blocked?
- When it tries to enter a synchronized block/method that's already locked by another thread.
✅ Example Blocked State
class Shared {
synchronized void print() {
System.out.println(Thread.currentThread().getName() + " is printing...");
try { Thread.sleep(1000); } catch (Exception e) {}
}
}
class MyThread extends Thread {
Shared shared;
MyThread(Shared s) { this.shared = s; }
public void run() {
shared.print(); // May enter Blocked state if lock is taken
}
}
public class Main {
public static void main(String[] args) {
Shared s = new Shared();
MyThread t1 = new MyThread(s);
MyThread t2 = new MyThread(s);
t1.start(); // Gets lock first
t2.start(); // Will be Blocked until t1 releases the lock
}
}
🧠 Key Notes:
- Blocked ≠ Waiting — Blocked means waiting for a lock, not just sleeping or paused.
- As soon as the lock is released, the blocked thread goes back to the Runnable state.
Waiting vs Timed Waiting
In Java, threads can pause execution voluntarily, without being blocked by another thread. These pauses fall into two categories:
🔄 Waiting State
- A thread is in the Waiting state when it waits indefinitely for another thread to notify it.
- It can be resumed only by
notify()
ornotifyAll()
.
✅ Example: Waiting
synchronized(obj) {
obj.wait(); // Thread enters Waiting state
}
- Use: Inter-thread communication (e.g., producer-consumer problems)
⏱️ Timed Waiting State
- A thread waits for a limited amount of time, then automatically resumes.
- Used when the thread should not wait forever.
✅ Common methods that cause Timed Waiting:
Thread.sleep(time)
join(time)
wait(time)
LockSupport.parkNanos()
✅ Example: Timed Waiting
Thread.sleep(2000); // Sleeps for 2 seconds
Thread.sleep(), join(), and yield() Methods
These are important thread control methods in Java that influence the execution timing and coordination between threads.
💤 1. Thread.sleep(milliseconds)
- Pauses the current thread for a specified time.
- The thread moves to the Timed Waiting state.
- Does not release locks it holds.
✅ Example:
try {
Thread.sleep(1000); // Sleep for 1 second
} catch (InterruptedException e) {
e.printStackTrace();
}
🔗 2. join()
- Used to wait for another thread to finish.
- The calling thread goes into Waiting or Timed Waiting state.
- Ensures one thread completes before another continues.
✅ Example:
Thread t1 = new Thread(() -> {
System.out.println("Thread 1 is running...");
});
t1.start();
t1.join(); // Main thread waits for t1 to finish
System.out.println("Main thread continues after t1");
🔄 3. yield()
- Suggests that the current thread pause to let other threads of equal priority run.
- Moves the thread back to Runnable state.
- Not guaranteed — it's a hint to the scheduler.
✅ Example:
Thread.yield(); // Hints scheduler to switch threads
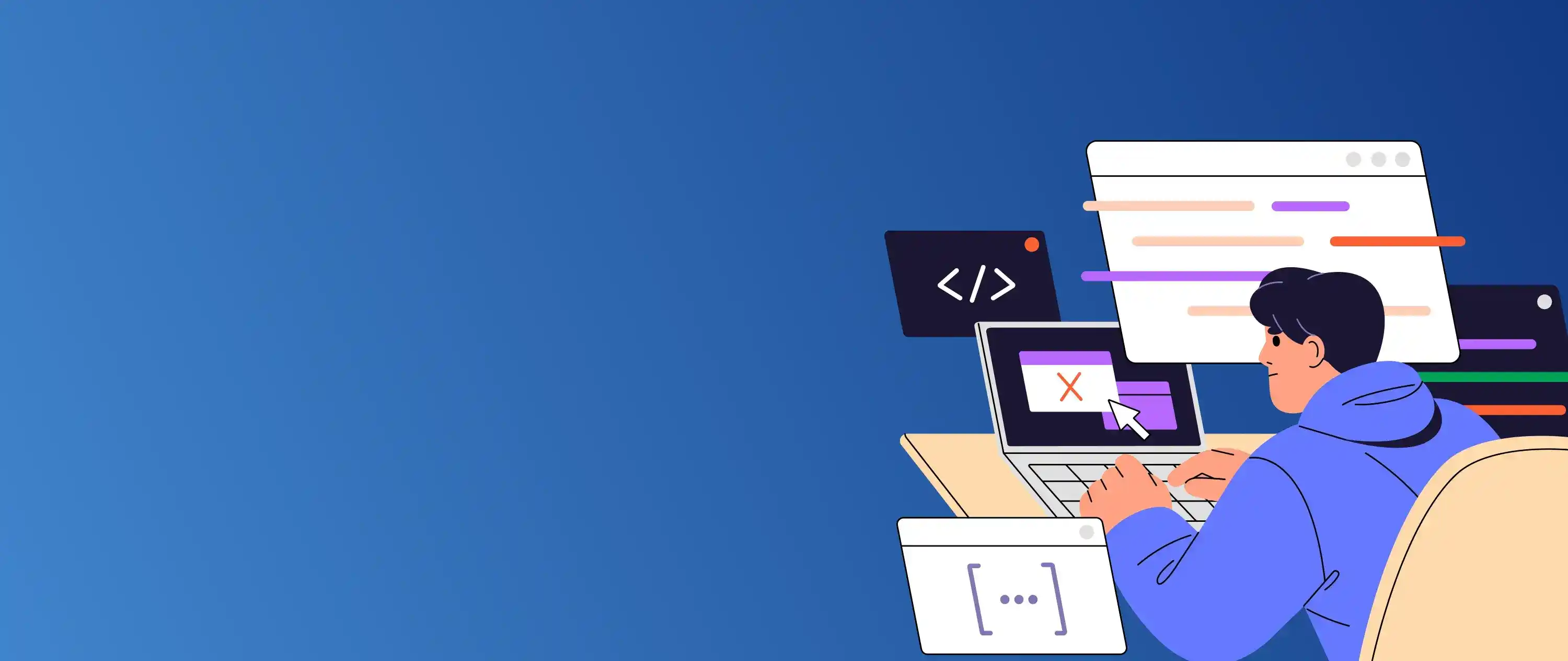
Need help for Hire Java Developers?
Thread Synchronization and Locks
When multiple threads access shared resources, they can cause data inconsistency if not properly synchronized. Synchronization ensures that only one thread at a time accesses critical code sections.
🔐 Why Synchronization?
Without synchronization, threads may:
- Read/write shared variables at the same time
- Cause race conditions
- Produce unpredictable results
✅ 1. Synchronized Methods
Locks the entire method — only one thread can access it at a time for a given object.
class Counter {
private int count = 0;
public synchronized void increment() {
count++;
}
}
✅ 2. Synchronized Blocks
Locks only a specific block of code, giving better control and performance.
class Counter {
private int count = 0;
private final Object lock = new Object();
public void increment() {
synchronized(lock) {
count++;
}
}
}
🔐 Intrinsic Locks (Monitor Locks)
Every object in Java has a built-in lock (monitor). When a thread enters a synchronized method/block, it acquires that object's lock.
✅ 3. Static Synchronization
Used when threads share static data across instances.
public static synchronized void syncStaticMethod() {
// Locked on the class object, not instance
}
⚠️ Important:
- Synchronization can lead to blocked threads.
- Always avoid unnecessary synchronization to improve performance.
Thread Priority and Scheduling
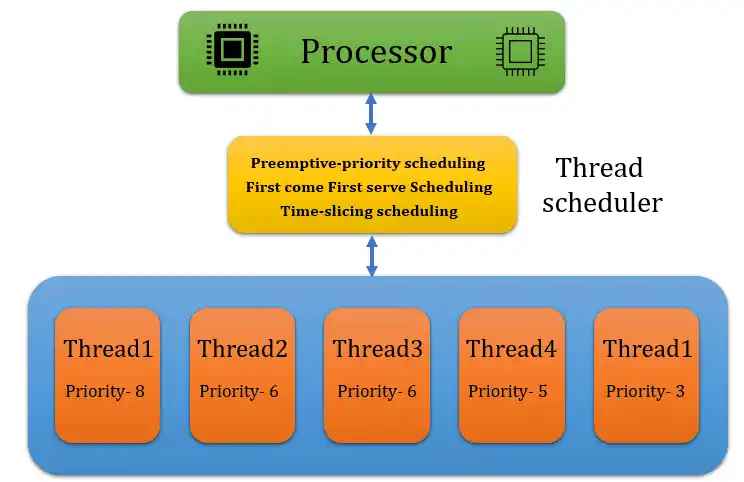
Image Source: google
Thread Priority | Thread Scheduling |
---|---|
Defines the importance level of a thread | Determines the order in which threads are executed |
Set by the programmer or system | Managed by the operating system or scheduler |
Higher priority threads are preferred for execution | Depends on algorithm (e.g., Round Robin, FCFS, Priority) |
Does not decide when the thread runs | Actually controls execution timing of threads |
Helps in preemptive scheduling decisions | Uses priority along with other factors for execution |
May cause starvation of low-priority threads | Tries to ensure fair CPU time among threads |
Dead State Thread Termination
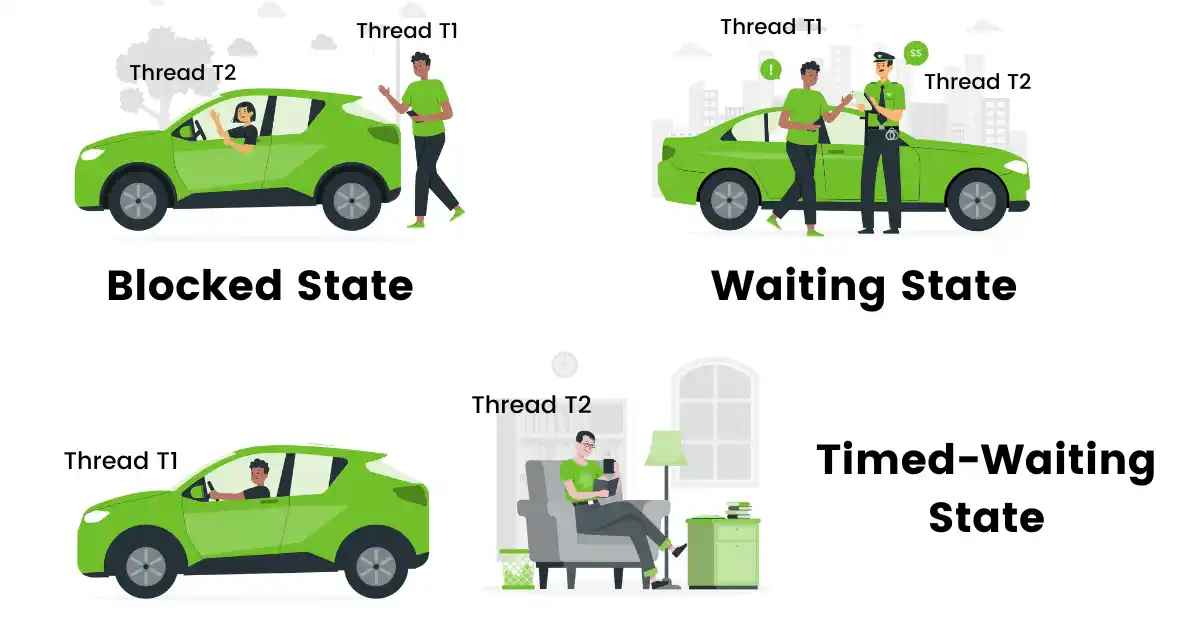
Image Source: google
When working with threads in programming, particularly in languages like Java or Python, understanding the dead state and how threads terminate is important for proper thread lifecycle management.
🔚 Dead State (Thread Termination)
The dead state is the final state of a thread's lifecycle. A thread enters this state when it finishes execution or is forcefully terminated.
🔁 Thread Lifecycle Overview (Quick Recap)
- New – Thread is created.
- Runnable – Ready to run, waiting for CPU.
- Running – Actively executing.
- Blocked/Waiting – Paused, waiting for a resource or condition.
- Terminated (Dead) – Execution finished or stopped.
✅ Causes of Thread Termination
- The
run()
method completes normally. - An uncaught exception occurs in the thread.
- The thread is forcefully stopped (not recommended, e.g., using
stop()
in Java). - In some systems, the main program ends, and background threads terminate.
📌 Key Points About Dead State
- Once a thread is in the dead state, it cannot be restarted.
- Trying to call
start()
on a dead thread will cause a runtime error. - Resources used by the thread are released once it terminates.
📋 Example in Java:
Thread t = new Thread(() -> {
System.out.println("Thread is running...");
});
t.start(); // Thread enters Running state
t.join(); // Wait for thread to finish -> Dead state
System.out.println("Thread has terminated.");
🧾 Summary Table
✅ Facts | ❌ Restrictions |
---|---|
Thread is in dead state after completion or termination | Cannot restart a dead thread |
Resources are released after termination | No more code execution possible in dead thread |
Thread may die due to error, completion, or force stop | Using deprecated stop methods can be unsafe |
Final stage of a thread lifecycle | No built-in way to revive a dead thread |
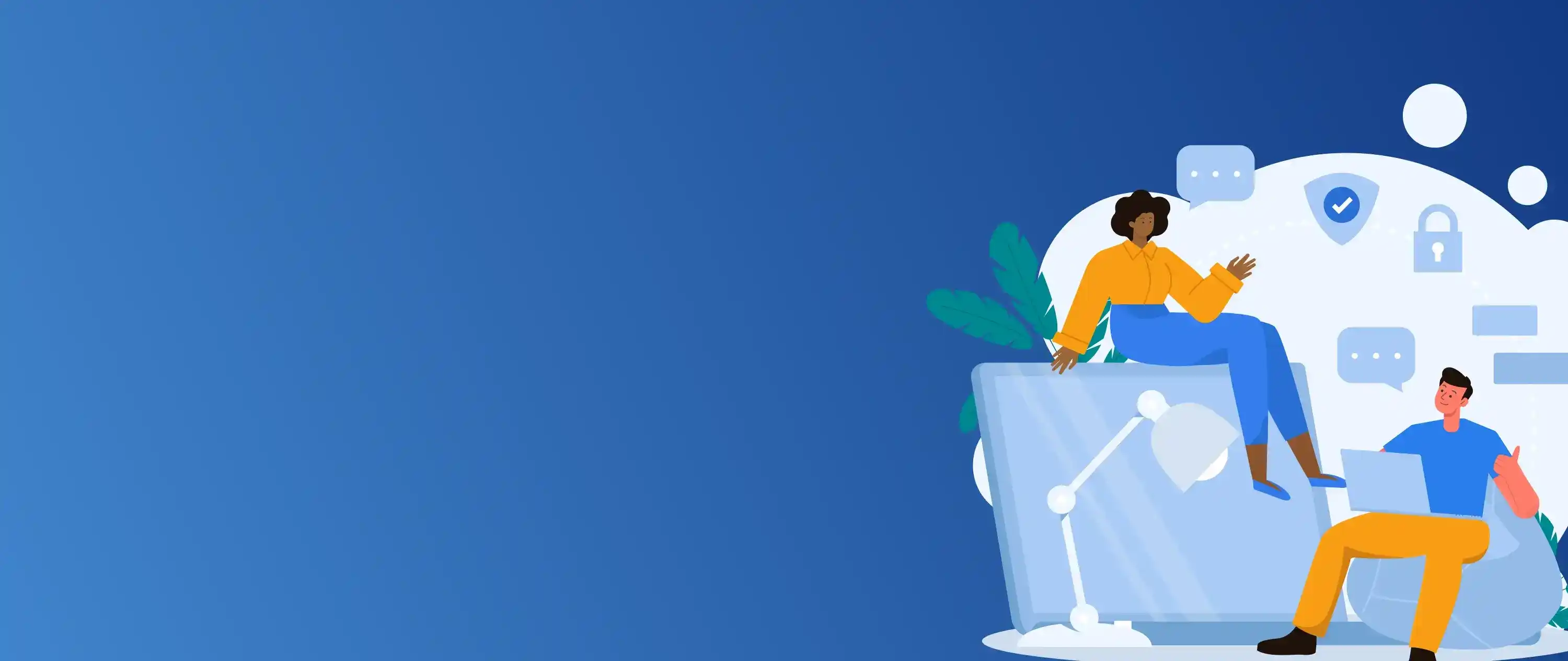
Do You Want to Hire Expert Java Developers?
Thread Management Best Practices
✅ Best Practice | 💡 Benefit |
---|---|
Limit number of threads | Prevents CPU overload and resource contention |
Use thread pools | Improves performance and reuses resources efficiently |
Avoid blocking operations | Reduces thread idle time and improves responsiveness |
Handle exceptions inside threads | Prevents unexpected crashes |
Use synchronization carefully | Avoids deadlocks and race conditions |
Name your threads | Easier debugging and logging |
Use high-level concurrency APIs | Simplifies thread management and increases reliability |
Monitor thread activity | Helps identify performance issues and bugs |
Shutdown threads gracefully | Prevents resource leaks and ensures safe termination |
Avoid deprecated methods | Ensures safe and future-proof code |
FAQs
Q.1. What is the New state in a thread's life cycle?
A : A thread is created but not started yet.
Q.2. What happens when a thread moves to Runnable?
A : It is ready to run and waiting for CPU time.
Q.3. What is the Running state?
A : The thread is actively executing its code.
Q.4. When does a thread enter the Blocked state?
A : When it waits for a resource like a lock or I/O.
Q.5. What is the Dead state in a thread's life cycle?
A : The thread has finished execution or is terminated.
Q.6. Can a thread be restarted after it dies?
A : No, a dead thread cannot be restarted.
Q.7. How does a thread go from New to Runnable?
A : By calling the start()
method.
Q.8. How does a thread go from Runnable to Running?
A : When the scheduler picks it for execution.
Q.9. How can a thread leave the Blocked state?
A : When the resource it needs becomes available.
Q.10. What causes a thread to terminate?
A : It finishes its task or an exception occurs.